728x90
반응형
Conv2D-Conv2D-MaxPooling2D
import tensorflow as tf
mnist=tf.keras.datasets.fashion_mnist
(x_train, y_train),(x_test, y_test)=mnist.load_data()
x_train, x_test = x_train / 255.0, x_test / 255.0
x_train = x_train.reshape((60000, 28, 28, 1))
x_test = x_test.reshape((10000, 28, 28, 1))
model = tf.keras.Sequential([
tf.keras.layers.InputLayer(input_shape=(28,28,1)),
tf.keras.layers.Conv2D(32,(3,3),activation='relu', padding='same'),
tf.keras.layers.Conv2D(64,(3,3),activation='relu', padding='same'),
tf.keras.layers.MaxPool2D((2,2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(128,activation='relu'),
tf.keras.layers.Dense(10,activation='softmax')
])
model.summary()
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
model.fit(x_train, y_train, epochs=5)
model.evaluate(x_test, y_test)
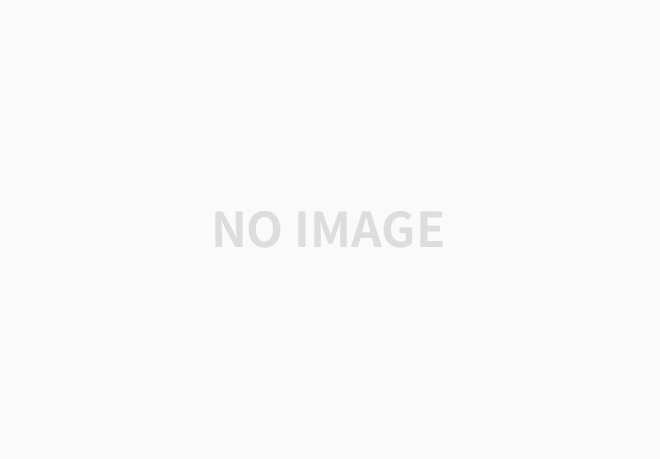
- 1차 합성곱(conv2d)의 가중치는 3*3*1*32=288의 크기이며 편향은 가중치의 갯수만큼 필요하여 32개가 됩니다.
3*3*1*32+32=320
- 2차 합성곱(conv3d)의 가중치는 3*3*32*64=18432의 크기이며 편향의 가중치의 갯수만큼 필요하여 64개가 됩니다.
3*3*1*64+64=18496
- Flatten 층은 완전 연경 층의 입력층으로 14*14*64=12544 노드로 구성됩니다.
- 은닉층의 노트는 128개, Flatten 층과 은닉층 연결에 필요한 가중치는 12544*128=1605632, 편향은 가중치의 갯수만큼 필요하여 128개가 됩니다
12544*128+128=1605760
- 출력층의 노드는 10개로 은닉층과 출력층 연결에 필요한 가중치의 갯수는 128*10, 편향은 가중치의 갯수만큼 필요하여
10개가 됩니다.
128*10+10=1290
학습의 대상이 되는 매개변수의 총 갯수는 320+18496+1605760+1290=1625866이 됩니다.
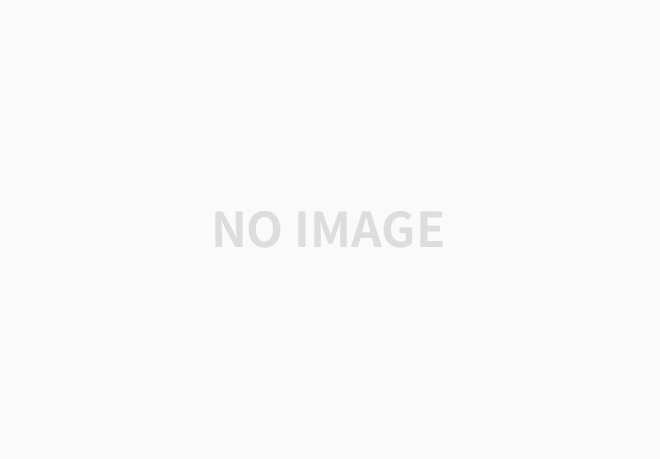
Conv2D-MaxPooling2D
import tensorflow as tf
mnist=tf.keras.datasets.fashion_mnist
(x_train, y_train),(x_test, y_test)=mnist.load_data()
x_train, x_test = x_train / 255.0, x_test / 255.0
x_train = x_train.reshape((60000, 28, 28, 1))
x_test = x_test.reshape((10000, 28, 28, 1))
model = tf.keras.Sequential([
tf.keras.layers.InputLayer(input_shape=(28,28,1)),
tf.keras.layers.Conv2D(32,(3,3),activation='relu', padding='same'),
tf.keras.layers.MaxPool2D((2,2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(10,activation='softmax')
])
model.summary()
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
model.fit(x_train, y_train, epochs=5)
model.evaluate(x_test, y_test)
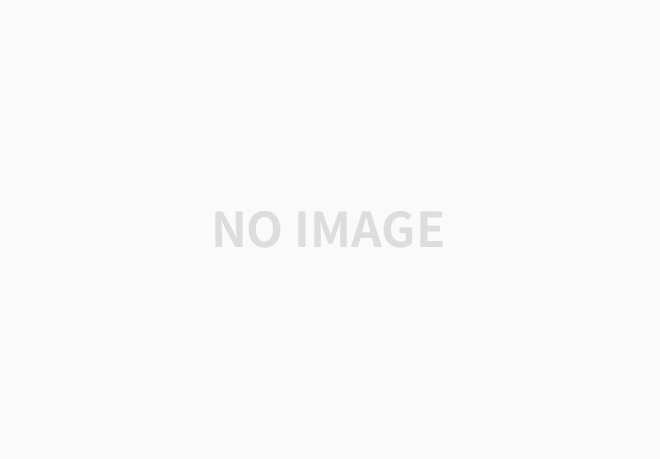
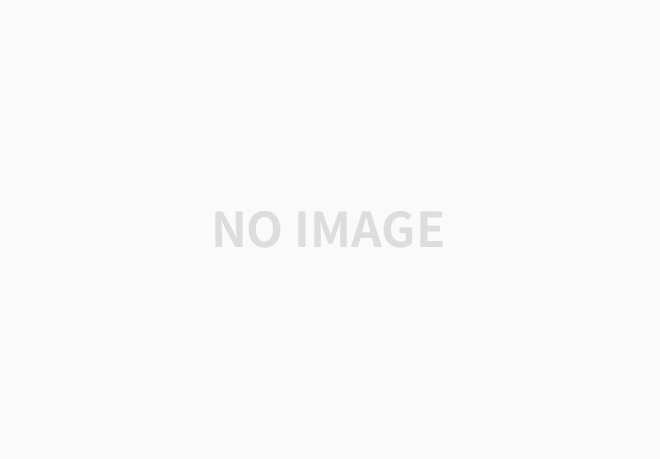
728x90
반응형
'즐거운프로그래밍' 카테고리의 다른 글
[딥러닝] CNN 활용 예제(엔비디아 자료 보고 신경망 구성하기) (1) | 2023.11.01 |
---|---|
[딥러닝] CNN 활용 맛보기 2 (0) | 2023.11.01 |
[딥러닝] 6x6x2, 6x6x3 입력 이미지의 합성곱과 필터 늘리기 (0) | 2023.11.01 |
[딥러닝] 3x3x2 입력 이미지의 합성곱 (0) | 2023.11.01 |
[딥러닝] 필터 역할 살펴보기 - 연습문제 (0) | 2023.11.01 |